Web animations have become a crucial element in enhancing user experience and making websites more interactive and engaging. JavaScript, being one of the most popular programming languages for web development, offers numerous ways to create animations.
JavaScript animation is a powerful tool for web developers. It helps create engaging, interactive, and visually appealing web experiences. By understanding the basics of how JavaScript manipulates web elements to create animations, developers can choose the most appropriate method for their projects
How JavaScript Animations Work
JavaScript animations work by progressively changing the properties of HTML and CSS elements over time. This can include changes in size, position, color, opacity, and other properties that can be animated. JavaScript executes these changes at runtime, allowing for interactive and conditional animations based on user inputs like mouse clicks, scrolling, or keyboard actions.
Why Animate with JavaScript?
Animations can make your website feel more dynamic and alive. Here's why you should consider using JavaScript for animations:
Interactivity: JavaScript allows animations to respond to user actions like clicks, mouse movements, and keypresses, making the website more interactive.
Control: It provides detailed control over animation sequences, timing, and synchronization between different animated elements.
Compatibility: JavaScript works across all modern browsers and devices, ensuring your animations reach a wide audience.
Performance: With advancements in JavaScript engines and the development of APIs like ‘requestAnimationFrame’, JavaScript animations can be optimized for high performance.
Types of JavaScript Animations
CSS Transitions & Animations: For simple animations, JavaScript can be used to add or remove CSS classes to elements, triggering CSS transitions or animations.
JavaScript Animation Libraries: Libraries like Three.js, GSAP (GreenSock Animation Platform), and Anime.js offer powerful and easy-to-use tools to create complex animations.
Canvas Animations: The HTML5 <canvas> element, manipulated via JavaScript, is perfect for creating 2D and 3D graphics and animations.
SVG Animations: JavaScript can manipulate Scalable Vector Graphics (SVG) for creating resolution-independent animations, ideal for logos and illustrations.
WebGL: For high-performance 2D and 3D animations, WebGL (Web Graphics Library) can be used with JavaScript to render graphics in any modern web browser.
Methods of Implementing JavaScript Animations
CSS Transitions and Animations Triggered by JavaScript: JavaScript can dynamically add or remove CSS classes from elements, triggering CSS transitions or animations. This method is useful for simple animations and leverages the power of CSS for the visual effects.
Direct Manipulation with JavaScript: For more control and complex animations, JavaScript can directly alter styles and attributes of elements in real-time. The ‘requestAnimationFrame’ API is commonly used for this purpose, as it provides a more efficient and smoother animation loop than traditional ‘setInterval’ or ‘setTimeout’ methods.
SVG and Canvas Animations: JavaScript can manipulate SVG (Scalable Vector Graphics) and canvas elements to create resolution-independent and complex graphical animations. This is especially useful for drawing applications, games, and interactive infographics.
Web Animation API: A modern approach to animations in JavaScript, this API provides a more powerful and flexible way to create complex animations directly in JavaScript, without relying heavily on CSS.
Animation Libraries: There are numerous JavaScript libraries designed to simplify the creation of animations. Libraries like GreenSock Animation Platform (GSAP), Anime.js, and Velocity.js offer advanced capabilities, cross-browser compatibility, and optimization.
Getting Started with JavaScript Animation
To begin animating with JavaScript, you'll need a basic understanding of HTML, CSS, and JavaScript. If you're new to web development, it's recommended to familiarize yourself with these technologies first. Once you're comfortable with the fundamentals, you can start experimenting with JavaScript animation.
Here's a simple example to get you started:
HTML
CSS
JavaScript
In this example, the ‘<div>’ element with the ID ‘animateMe’ will move to the right by changing its ‘left’ property over time, creating a simple animation effect.
Best Practices for JavaScript Animation
Use ‘requestAnimationFrame’ for better performance: It allows the browser to optimize the animation, leading to smoother animations and reduced power consumption.
Keep it simple: Excessive animations can be distracting. Aim for subtlety unless the design specifically requires more.
Test across devices: Ensure your animations perform well on various devices, especially mobile devices where performance can vary.
Consider accessibility: Provide options to reduce motion for users who are sensitive to animations.
Here's a beginner-friendly YouTube tutorial on JavaScript animation to walk through a basic example. By following along, you'll grasp the foundational concepts needed to craft intricate and captivating web animations.
JavaScript animation opens up a world of possibilities for creating dynamic and engaging web experiences. While this guide covers the basics, there's much more to explore. If you're eager to dive deeper, consider enrolling in our Front End Fundamentals course, available for lifetime access or through Covalence+.
As you continue your journey into JavaScript animation, don't be afraid to experiment, learn from tutorials and documentation, and unleash your creativity to bring your web projects to life. Happy animating!
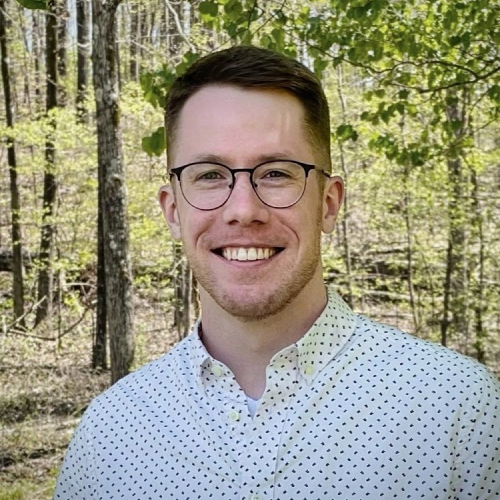