React.js, often just called React, has soared in popularity as one of the go-to JavaScript libraries for building dynamic and responsive user interfaces. Developed by Facebook, React's declarative, component-based approach makes it an efficient and flexible choice for developers. It is also renowned for its exceptional Developer Experience (DX), which is one of the key reasons it has become so widely adopted. Whether you're building a small interactive widget or a large-scale application, React can help you create a seamless experience. Here's a beginner's guide to getting started with React.
Understanding React:
React introduces the concept of the Virtual Document Object Model (VDOM), which helps keep the browser’s DOM in sync with the application’s state. This makes React incredibly efficient, as updates can be made quickly and precisely. State, a general programming concept, is integral in React and refers to the data that determines a component’s rendering and behavior. Whenever state changes occur, React will efficiently update the necessary components in the VDOM and minimize the changes needed in the actual DOM. This results in incredible performance and a smooth user experience. At its core, React operates on components, which are small, reusable pieces of code that represent parts of the user interface.
Why Choose React?
Component-Based Architecture:
React’s modular structure encourages reusability of code, making it easier to manage and scale your project.
Declarative UI:
React makes it straightforward to create interactive UIs. Design simple views for each state in your application, and React will efficiently update and render the right components when your data changes.
Rich Ecosystem:
From state management tools like Redux to routing solutions like React Router, React boasts a vast ecosystem. This ecosystem provides libraries and tools to meet virtually any need your application might have.
Strong Community Support:
Being one of the most popular front-end libraries means there's a large community of developers. You'll find plenty of resources, from tutorials to forums, where you can learn and get help.
It’s Fun:
Don’t overlook how enjoyable it is to use and write code with React!
Setting Up Your First React Project
Prerequisites
Before you begin, make sure you have Node.js installed on your computer. Node.js comes with npm (Node Package Manager), which you'll use to create and manage your React project. You can download and install the latest Long Term Support (LTS) version of Node.js from the official website, or use a package manager for your operating system:
- Windows: Use Chocolatey to install Node.js.
- Mac/Linux: Use Curl with NVM (Node Version Manager) to install Node.js.
Create React App
Many seasoned developers are familiar with the trusted tool for learning React—Create React App (CRA). However, as of March 2023, CRA has been deprecated. React’s official documentation has removed references to it, and the CRA project will no longer be maintained. While it's still usable, there are now modern alternatives to replace our old friend that was put out to pasture.
For more information, you can visit React’s documentation on starting a new React project, but we will be taking a different approach!
Vite
While CRA may be the old busted, Vite has entered the fray as our new hotness. It is a modern and efficient alternative for setting up React projects that, while not specifically listed by the official documentation, is growing in popularity on Stack Overflow posts, online articles, and Reddit threads. Vite, which means "quick" in French, lives up to its name by offering a lightning-fast development environment. Here are some reasons why Vite is an excellent choice:
- Fast Startup and Hot Module Replacement (HMR): Vite's architecture allows for incredibly fast startup times and near-instant HMR. Buttery smooth.
- Out-of-the-Box Support: Vite provides built-in support for JavaScript, TypeScript, JSX, and CSS Modules, along with many other modern web technologies. This reduces the need for additional configuration.
- Optimized Build: Vite uses Rollup for production builds, offering a highly optimized and efficient build process.
- Modern Features: Vite supports ES modules natively. Neat!
Open your terminal and run:
Or you can simply type:
Then just follow the prompts in your terminal.
This will create a new directory called my-first-react-app with all the necessary files and dependencies. The npm run dev command runs the app in development mode, provides a port number on localhost, and all you need to do is visit it in your browser of choice.
Understanding the Basics
JSX: The Syntax of React
JSX (JavaScript XML) allows you to write HTML elements directly in JavaScript and place them in the DOM without using functions like createElement or appendChild. This makes it easy for developers who are already familiar with HTML to seamlessly integrate it into their JavaScript code. It's like magic!
Example of JSX:
Components
Components are the heart of React applications. They let you split the UI into independent, reusable pieces, each encapsulating its own structure, logic, and style. This modular approach allows you to build complex UIs from small, isolated components. You’ve already seen a component just above, actually! In modern React development, components are typically just JavaScript functions.
Example of a Component:
State and Props
Props are arguments passed into React components as an object, allowing you to customize how they behave or render. They are passed to components via HTML attributes and can be thought of like function arguments.
Example of Props:
State is a built-in object that allows components to store and manage dynamic data. It enables components to react and re-render in response to changes, such as user input or data fetching. Essentially, think of it as a way for your component to “remember” some information and display it. For example, you might want to count the number of times a button is clicked.
Example of State:
You’ll get two things from useState: the current state, and the function that lets you update it. You can give them any names, but the convention is to write [something, setSomething].
Back to the Project
In our Vite projects, there is a src folder where all of our code will go. It will be filled with the introductory boilerplate provided by the bootstrapped Vite project. Let’s delete every file and re-write the minimum necessary to learn how this is pieced together.
- Delete all the contents from the src folder, but leave the folder itself.
- Create a file called main.jsx in the src folder (note: this is Vite’s requirement similar to CRA’s old index.jsx entry point).
- You’ll need to add the following into it: React, ReactDOM, our-yet-to-exist App component, and then to tell our project it will find a root element in the DOM and render our App (which represents the entire application) component into it.
- Next we create our App.jsx file. It is a common convention to uppercase the file name to match the component name found within the file.
- You’ll add the simplest component possible into it.
- Confirm your terminal is running the project, if not, make sure to be in the correct directory and run the npm run dev command.
- Navigate to the provided port in your browser, e.g. http://localhost:5173/
- Bask in glory!
Expanding Your Knowledge
Once you're comfortable with the basics, you can explore more advanced topics, such as:
- Hooks: Introduced in React 16.8, hooks allow you to use state and other React features in functional components.
- Context API: A way to share values like themes and user data between components without having to explicitly pass props through every level of the tree.
- React Router: A library to handle routing in your single-page application, making navigation between views smooth and easy.
React.js offers a potent, component-based approach to web application development. Armed with the fundamentals—JSX, components, props, and state—you're ready to create dynamic and responsive web applications. Remember, the React ecosystem is vast and evolving, offering endless opportunities for learning and innovation.
Ready to take your React skills to the next level? Dive deeper into React with Covalence's advanced React course, the only resource you'll ever need to become a React expert. Gain access for life when you purchase the course, or pay-as-you-go when you subscribe to Covalence+ to learn React - and everything else we teach - for an all-inclusive learning experience.
Don't forget to explore Covalence's YouTube playlist, packed with helpful tutorials on React.js to complement your learning journey.
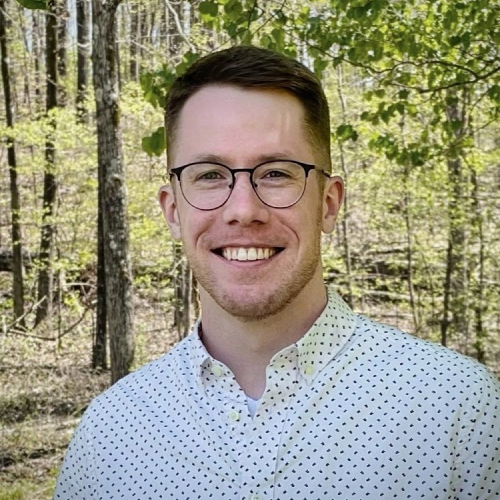